Elastic Email Perl API Library
Introduction
When you integrate Elastic Email with your application, you can interact with your Elastic Email account directly by our API. You can easily integrate your email flow, manage your contacts and templates, send emails, and track email statistics directly from your app.
This page will help you easily integrate with Elastic Email using the Perl library. You can find our whole downloadable Perl repository on GitHub. You can also use our comprehensive API documentation.
Elastic Email API v4 uses REST architecture, which allows you to perform various code actions, including directly through your app.
The maximum email size of your entire message or message + attachment cannot exceed 20MB.
The attachment format is the file’s content as a byte array or a Base64 string.
The maximum number of recipients has no limit for one campaign. It depends on the pricing plan.
The API has a limit of 20 concurrent connections and a hard timeout of 600 seconds per request.
On this page, you will find how to authenticate your application and what the requirements for the integration are. You will be also provided with a quick start guide on how to start using API, followed by code samples.
Authentication
To provide valid authentication and start using our API, you will need an API key. To generate your API key, enter settings on your Elastic Email account and go to Settings -> Manage API Keys -> Create or you can also click on the link:
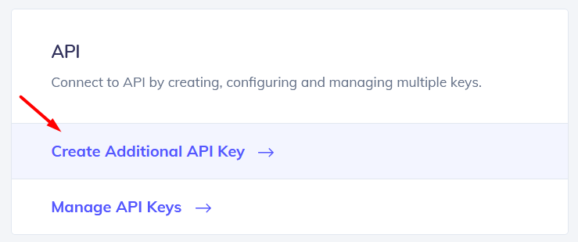
At this point, you can set custom permissions and optional access for your API key.
Security tip: The restriction forces the API Key to work only with an IP or IP range that will be specified in this field.
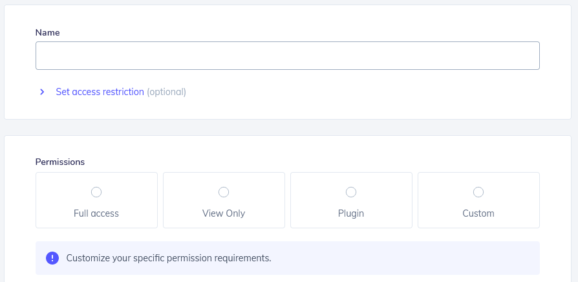
Once you create your API key, keep it safe as it will be used for every API call you make in order to identify you and confirm your account’s credentials. You can create either up to 15 or an unlimited amount of API keys based on your pricing plan.
Your API key should be sent inside the header with the parameter name ‘x-elasticemail-apikey’ and your API key as a value.
Installation and Usage
Requirements
Use cpanm to install the module dependencies:
cpanm --local-lib=~/perl5 local::lib && eval $(perl -I ~/perl5/lib/perl5/ -Mlocal::lib) cpanm --quiet --no-interactive Class::Accessor Test::Exception Test::More Log::Any LWP::UserAgent URI::Query Module::Runtime DateTime Module::Find Moose::Role JSON
Installation
Our official downloadable Perl library is available on GitHub.
To load the API packages, put the following lines:
use ElasticEmail::CampaignsApi; use ElasticEmail::ContactsApi; use ElasticEmail::EmailsApi; use ElasticEmail::EventsApi; use ElasticEmail::FilesApi; use ElasticEmail::InboundRouteApi; use ElasticEmail::ListsApi; use ElasticEmail::SecurityApi; use ElasticEmail::SegmentsApi; use ElasticEmail::StatisticsApi; use ElasticEmail::SubAccountsApi; use ElasticEmail::SuppressionsApi; use ElasticEmail::TemplatesApi; use ElasticEmail::VerificationsApi;
To load the models, put the following lines:
use ElasticEmail::Object::AccessLevel; use ElasticEmail::Object::AccountStatusEnum; use ElasticEmail::Object::ApiKey; use ElasticEmail::Object::ApiKeyPayload; use ElasticEmail::Object::BodyContentType; use ElasticEmail::Object::BodyPart; use ElasticEmail::Object::Campaign; use ElasticEmail::Object::CampaignOptions; use ElasticEmail::Object::CampaignRecipient; use ElasticEmail::Object::CampaignStatus; use ElasticEmail::Object::CampaignTemplate; use ElasticEmail::Object::ChannelLogStatusSummary; use ElasticEmail::Object::CompressionFormat; use ElasticEmail::Object::ConsentData; use ElasticEmail::Object::ConsentTracking; use ElasticEmail::Object::Contact; use ElasticEmail::Object::ContactActivity; use ElasticEmail::Object::ContactHistEventType; use ElasticEmail::Object::ContactHistory; use ElasticEmail::Object::ContactPayload; use ElasticEmail::Object::ContactSource; use ElasticEmail::Object::ContactStatus; use ElasticEmail::Object::ContactUpdatePayload; use ElasticEmail::Object::ContactsList; use ElasticEmail::Object::DeliveryOptimizationType; use ElasticEmail::Object::EmailContent; use ElasticEmail::Object::EmailData; use ElasticEmail::Object::EmailMessageData; use ElasticEmail::Object::EmailRecipient; use ElasticEmail::Object::EmailSend; use ElasticEmail::Object::EmailStatus; use ElasticEmail::Object::EmailTransactionalMessageData; use ElasticEmail::Object::EmailValidationResult; use ElasticEmail::Object::EmailValidationStatus; use ElasticEmail::Object::EmailView; use ElasticEmail::Object::EmailsPayload; use ElasticEmail::Object::EncodingType; use ElasticEmail::Object::EventType; use ElasticEmail::Object::EventsOrderBy; use ElasticEmail::Object::ExportFileFormats; use ElasticEmail::Object::ExportLink; use ElasticEmail::Object::ExportStatus; use ElasticEmail::Object::FileInfo; use ElasticEmail::Object::FilePayload; use ElasticEmail::Object::FileUploadResult; use ElasticEmail::Object::InboundPayload; use ElasticEmail::Object::InboundRoute; use ElasticEmail::Object::InboundRouteActionType; use ElasticEmail::Object::InboundRouteFilterType; use ElasticEmail::Object::ListPayload; use ElasticEmail::Object::ListUpdatePayload; use ElasticEmail::Object::LogJobStatus; use ElasticEmail::Object::LogStatusSummary; use ElasticEmail::Object::MergeEmailPayload; use ElasticEmail::Object::MessageAttachment; use ElasticEmail::Object::MessageCategory; use ElasticEmail::Object::NewApiKey; use ElasticEmail::Object::NewSmtpCredentials; use ElasticEmail::Object::Options; use ElasticEmail::Object::RecipientEvent; use ElasticEmail::Object::Segment; use ElasticEmail::Object::SegmentPayload; use ElasticEmail::Object::SmtpCredentials; use ElasticEmail::Object::SmtpCredentialsPayload; use ElasticEmail::Object::SortOrderItem; use ElasticEmail::Object::SplitOptimizationType; use ElasticEmail::Object::SplitOptions; use ElasticEmail::Object::SubAccountInfo; use ElasticEmail::Object::SubaccountEmailCreditsPayload; use ElasticEmail::Object::SubaccountEmailSettings; use ElasticEmail::Object::SubaccountEmailSettingsPayload; use ElasticEmail::Object::SubaccountPayload; use ElasticEmail::Object::SubaccountSettingsInfo; use ElasticEmail::Object::SubaccountSettingsInfoPayload; use ElasticEmail::Object::Suppression; use ElasticEmail::Object::Template; use ElasticEmail::Object::TemplatePayload; use ElasticEmail::Object::TemplateScope; use ElasticEmail::Object::TemplateType; use ElasticEmail::Object::TransactionalRecipient; use ElasticEmail::Object::Utm; use ElasticEmail::Object::VerificationFileResult; use ElasticEmail::Object::VerificationFileResultDetails; use ElasticEmail::Object::VerificationStatus;
Put the Perl SDK under the 'lib' folder in your project directory, then run the following:
#!/usr/bin/perl use lib 'lib'; use strict; use warnings; # load the API package use ElasticEmail::CampaignsApi; use ElasticEmail::ContactsApi; use ElasticEmail::EmailsApi; use ElasticEmail::EventsApi; use ElasticEmail::FilesApi; use ElasticEmail::InboundRouteApi; use ElasticEmail::ListsApi; use ElasticEmail::SecurityApi; use ElasticEmail::SegmentsApi; use ElasticEmail::StatisticsApi; use ElasticEmail::SubAccountsApi; use ElasticEmail::SuppressionsApi; use ElasticEmail::TemplatesApi; use ElasticEmail::VerificationsApi; # load the models use ElasticEmail::Object::AccessLevel; use ElasticEmail::Object::AccountStatusEnum; use ElasticEmail::Object::ApiKey; use ElasticEmail::Object::ApiKeyPayload; use ElasticEmail::Object::BodyContentType; use ElasticEmail::Object::BodyPart; use ElasticEmail::Object::Campaign; use ElasticEmail::Object::CampaignOptions; use ElasticEmail::Object::CampaignRecipient; use ElasticEmail::Object::CampaignStatus; use ElasticEmail::Object::CampaignTemplate; use ElasticEmail::Object::ChannelLogStatusSummary; use ElasticEmail::Object::CompressionFormat; use ElasticEmail::Object::ConsentData; use ElasticEmail::Object::ConsentTracking; use ElasticEmail::Object::Contact; use ElasticEmail::Object::ContactActivity; use ElasticEmail::Object::ContactHistEventType; use ElasticEmail::Object::ContactHistory; use ElasticEmail::Object::ContactPayload; use ElasticEmail::Object::ContactSource; use ElasticEmail::Object::ContactStatus; use ElasticEmail::Object::ContactUpdatePayload; use ElasticEmail::Object::ContactsList; use ElasticEmail::Object::DeliveryOptimizationType; use ElasticEmail::Object::EmailContent; use ElasticEmail::Object::EmailData; use ElasticEmail::Object::EmailMessageData; use ElasticEmail::Object::EmailRecipient; use ElasticEmail::Object::EmailSend; use ElasticEmail::Object::EmailStatus; use ElasticEmail::Object::EmailTransactionalMessageData; use ElasticEmail::Object::EmailValidationResult; use ElasticEmail::Object::EmailValidationStatus; use ElasticEmail::Object::EmailView; use ElasticEmail::Object::EmailsPayload; use ElasticEmail::Object::EncodingType; use ElasticEmail::Object::EventType; use ElasticEmail::Object::EventsOrderBy; use ElasticEmail::Object::ExportFileFormats; use ElasticEmail::Object::ExportLink; use ElasticEmail::Object::ExportStatus; use ElasticEmail::Object::FileInfo; use ElasticEmail::Object::FilePayload; use ElasticEmail::Object::FileUploadResult; use ElasticEmail::Object::InboundPayload; use ElasticEmail::Object::InboundRoute; use ElasticEmail::Object::InboundRouteActionType; use ElasticEmail::Object::InboundRouteFilterType; use ElasticEmail::Object::ListPayload; use ElasticEmail::Object::ListUpdatePayload; use ElasticEmail::Object::LogJobStatus; use ElasticEmail::Object::LogStatusSummary; use ElasticEmail::Object::MergeEmailPayload; use ElasticEmail::Object::MessageAttachment; use ElasticEmail::Object::MessageCategory; use ElasticEmail::Object::NewApiKey; use ElasticEmail::Object::NewSmtpCredentials; use ElasticEmail::Object::Options; use ElasticEmail::Object::RecipientEvent; use ElasticEmail::Object::Segment; use ElasticEmail::Object::SegmentPayload; use ElasticEmail::Object::SmtpCredentials; use ElasticEmail::Object::SmtpCredentialsPayload; use ElasticEmail::Object::SortOrderItem; use ElasticEmail::Object::SplitOptimizationType; use ElasticEmail::Object::SplitOptions; use ElasticEmail::Object::SubAccountInfo; use ElasticEmail::Object::SubaccountEmailCreditsPayload; use ElasticEmail::Object::SubaccountEmailSettings; use ElasticEmail::Object::SubaccountEmailSettingsPayload; use ElasticEmail::Object::SubaccountPayload; use ElasticEmail::Object::SubaccountSettingsInfo; use ElasticEmail::Object::SubaccountSettingsInfoPayload; use ElasticEmail::Object::Suppression; use ElasticEmail::Object::Template; use ElasticEmail::Object::TemplatePayload; use ElasticEmail::Object::TemplateScope; use ElasticEmail::Object::TemplateType; use ElasticEmail::Object::TransactionalRecipient; use ElasticEmail::Object::Utm; use ElasticEmail::Object::VerificationFileResult; use ElasticEmail::Object::VerificationFileResultDetails; use ElasticEmail::Object::VerificationStatus; # for displaying the API response data use Data::Dumper; my $api_instance = ElasticEmail::CampaignsApi->new( # Configure API key authorization: apikey api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, # uncomment below to setup prefix (e.g. Bearer) for API key, if needed #api_key_prefix => {'X-ElasticEmail-ApiKey' => 'Bearer'}, ); my $name = "name_example"; # string | Name of Campaign to delete eval { $api_instance->campaigns_by_name_delete(name => $name); }; if ($@) { warn "Exception when calling CampaignsApi->campaigns_by_name_delete: $@\n"; }
Quick start guide
In this section, we will tell you the steps to start sending emails with our email API.
1. Register a free account
Register and activate your Elastic Email account to get access to our product.
2. Verify your domain
Follow the instructions on our settings page to verify your domain and start sending with us.
3. Create an API Key
Go to your setting to generate an API Key to add to your code later.
4. Install our libraries
Install our Perl library as explained in the Installation and usage section.
5. Send your first email with API
Now it’s time to send your first test email with API to make sure that everything is set up correctly after downloading the library and the authentication process.
my $email_message_data = ElasticEmail::Object::EmailMessageData->new(); # EmailMessageData | Email data my $recipient = ElasticEmail::Object::EmailRecipient->new(); $recipient->email("MyEmail "); my $content = ElasticEmail::Object::EmailContent->new(); my $body = ElasticEmail::Object::BodyPart->new(); $body->content('My test email content ;)'); my $body_content_type = 'HTML'; $body->content_type($body_content_type); $content->body([$body]); $content->reply_to('MyEmail '); $content->from('MyEmail '); $content->subject('Perl EE lib test'); $content->template_name('template name'); $email_message_data->recipients([$recipient]); $email_message_data->content($content); eval { my $result = $api_instance->emails_post(email_message_data => $email_message_data); print Dumper($result); }; if ($@) { warn "Exception when calling EmailsApi->emails_post: $@\n";
Code Samples
Once you have sent the test email with our API, you can start sending transactional emails and perform various operations directly through your app. We have prepared for you some code samples for most essential actions, including managing contacts and lists, creating templates, and sending and tracking emails. Thanks to them, your integration with our API will be an even easier and more pleasant experience.
In case any of the following snippets are changed in the meantime, the most recent version of this API Library is available on GitHub.
Managing Contacts
In this section, we will walk you through managing the contacts on your account using the Perl library.
Add Contacts
To add contacts to your account, you will need Access Level: ModifyContacts.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::ContactsApi; use ElasticEmail::Object::ContactPayload;
Generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ContactsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an array with new contacts.
You can pass an array with up to 1000 contacts.
The Email
field is mandatory, the rest is optional.
Find out more by checking our API's documentation.
my $contact_payload = ElasticEmail::Object::ContactPayload->new(); # ARRAY[ContactPayload] $contact_payload->email('test@domain.com'); $contact_payload->first_name('test'); $contact_payload->last_name('test'); $contact_payload->status('Active');
Specify an existing list name in options, otherwise, contacts will be added to all contacts.
my $listnames = ["perlList"]; # ARRAY[string] | Names of lists to which the uploaded contacts should be added to
And finally, call contacts_post
method from the API to add contacts:
my $result = $api_instance->contacts_post(contact_payload => @contact_payload_array, listnames => $listnames);
Add Bulk Contacts
Contacts can be also added in bulk. To add multiple contacts to your account, you will need Access Level: ModifyContacts.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::ContactsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ContactsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an instance of ContactsApi that will be used to add contacts.
my $list_name = "test"; # string | Name of an existing list to add these contacts to my $encoding_name = "ASCII"; # string | In what encoding the file is uploaded my $file = "./functions/files/contacts.csv"; # string $api_instance->contacts_import_post(list_name => $list_name, encoding_name => $encoding_name, file => $file);
Delete contacts
If you want to delete a contact, you will need Access Level: ModifyContacts.
To delete a contact, put the following code into your file.
Load library using the following line:
use ElasticEmail::ContactsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ContactsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an object with an array of contacts to delete.
Find out more by checking our API's documentation.
Create an instance of CampaignsApi that will be used to delete a campaign.
my $email = 'test@domain.com'; # string | Proper email address. $response = $apiInstance->contactsByEmailDelete($email);
And finally, call contacts_by_email_delete
method from the API to delete campaign:
$api_instance->contacts_by_email_delete(email => $email);
Export Contacts
If you want to export the selected contact to a downloadable file, you will need Access Level: Export.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::ContactsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ContactsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an options object:
- fileFormat - specify format in which file should be created, options are: "Csv" "Xml" "Json".
- emails - select contacts to export by providing array of emails
- fileName - you can specify file name of your choice
Other options:
- rule - eg.
rule=Status%20=%20Engaged
– Query used for filtering - compressionFormat - "None" "Zip"
Find out more by checking our API's documentation.
my $file_format = 'Csv'; # ExportFileFormats | Format of the exported file my $rule = 'Status = Engaged'; # string | Query used for filtering. my $compression_format = 'None'; # CompressionFormat | FileResponse compression format. None or Zip. my $file_name = 'Engaged Contacts.txt'; # string | Name of your file including extension.
And finally, call contacts_export_post
method from the API to export contacts:
my $result = $api_instance->contacts_export_post(file_format => $file_format, rule => $rule, compression_format => $compression_format, file_name => $file_name);
Managing Lists
In this section, we will walk you through managing your contact list on your account using the Perl library.
Add List
To add a list, you will need Access Level: ModifyContacts.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::Object::ListPayload; use ElasticEmail::ListsApi;
Get instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ListsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an object with details about the new list. Only ListName
is required.
You can also define if to allow unsubscription from the list and pass an email array of existing contacts on your account to add them to the list during list creation.
Find out more by checking our API's documentation.
my $list_payload = ElasticEmail::Object::ListPayload->new(); # ListPayload | $list_payload->list_name('List Name');
Create an instance of ListsApi that will be used to create a new list.
my $result = $api_instance->lists_post(list_payload => $list_payload);
Load List
To load a list, you will need Access Level: ViewContacts.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::ListsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ListsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
The only thing needed is a list name.
Find out more by checking our API's documentation.
my $name = 'your list name'; # string | Name of your list.
And finally, call lists_by_name_get
method from the API to load list details:
my $result = $api_instance->lists_by_name_get(name => $name);
Delete List
To remove a contact list from your account, you will need Access Level: ModifyContacts.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::ListsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::ListsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
The only thing needed is a list name.
Find out more by checking our API's documentation.
Create an instance of ListsApi that will be used to delete a list.
my $name = 'list name'; # string | Name of your list to delete. $response = $apiInstance->listsByNameDelete($name);
And finally, call lists_by_name_delete
method from the API to delete a list:
$api_instance->lists_by_name_delete(name => $name);
Creating Templates
An email template is a body of email prepared and saved under a given name. In this section, you will get to know how to add and load email templates.
Add Template
To add a template, you will need Access Level: ModifyTemplates.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::TemplatesApi; use ElasticEmail::Object::TemplatePayload; use ElasticEmail::Object::BodyPart;
Get client instance, generate and use your API key (remember to check the required access level):
my $api_instance = ElasticEmail::TemplatesApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an object with details about the new template:
- Name – the name of your template by which it can be identified and used
- Subject – specify the default subject for this template
- Body – specify actual body content eg. in HTML, PlainText or both
- TemplateScope – specify scope, "Personal" template won't be shared, "Global" template can be shared with your sub accounts.
Find out more by checking our API's documentation.
my $template_payload = ElasticEmail::Object::TemplatePayload->new(); # TemplatePayload | $template_payload->name('template name'); $template_payload->subject('template subject'); my $body = ElasticEmail::Object::BodyPart->new(); $body->content_type('HTML'); $body->content('<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" lang="EN"> <head> <style type="text/css"> .mydiv { background: #FFBD5A; text-align: center; padding: 40px; } </style> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> </head> <body> <div class="mydiv"> <h1>My template</h1> </div> </body> </html>'); $template_payload->template_scope('Global'); $template_payload->body([$body]);
And finally, call templatesPost
method from the API to create a template:
my $result = $api_instance->templates_post(template_payload => $template_payload);
Load Template
To load existing template details, you will need Access Level: ViewTemplates.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::TemplatesApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::TemplatesApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
To load a template, you need to specify its name:
Find out more by checking our API's documentation.
my $name = 'template name'; # string | Name of template.
And finally, call templates_by_name_get
method from the API to load a template:
my $result = $api_instance->templates_by_name_get(name => $name);
Delete Template
To delete a template, you will need Access Level: ModifyTemplates.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::TemplatesApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::TemplatesApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
To delete a template, you need to specify its name:
Find out more by checking our API's documentation.
my $name = 'template name'; # string | Name of template.
And finally, call templates_by_name_delete
method from the API to delete a template:
$api_instance->templates_by_name_delete(name => $name);
Sending Emails
In this section, we will tell you how you can start sending emails using the Perl library. You will get to know how to send bulk and transactional emails.
Send Transactional Emails
To send transactional emails, you will need Access Level: SendHttp.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::EmailsApi; use ElasticEmail::Object::TransactionalRecipient; use ElasticEmail::Object::EmailTransactionalMessageData; use ElasticEmail::Object::BodyPart; use ElasticEmail::Object::Options;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::EmailsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
First you need to specify email details:
- email recipients
- email content:
- body parts – in HTML, PlainText or in both
- from email – it needs to be your validated email address
- email subject
Find out more by checking our API's documentation.
my $email_transactional_message_data = ElasticEmail::Object::EmailTransactionalMessageData->new(); # EmailTransactionalMessageData | Email data my $recipient = ElasticEmail::Object::TransactionalRecipient->new(); $recipient->to(['test@domain.com']); $recipient->cc(['test@domain.com']); $recipient->bcc(['test@domain.com']); $email_transactional_message_data->recipients($recipient); my $content = ElasticEmail::Object::EmailContent->new(); my $body = ElasticEmail::Object::BodyPart->new(); $body->content('test'); my $body_content_type = 'HTML'; $body->content_type($body_content_type); $content->body([$body]); $content->reply_to('test@domain.com'); $content->from('test@domain.com'); $content->subject('template subject'); $content->template_name('template name'); my $options = ElasticEmail::Object::Options->new(); $options->channel_name('channel name'); $email_transactional_message_data->options($options); $email_transactional_message_data->content($content);
And finally, call emails_transactional_post
method from the API to send an email:
my $result = $api_instance->emails_transactional_post(email_transactional_message_data => $email_transactional_message_data);
Send Bulk Emails
Put the following code to your file to send a bulk email, meaning a single email sent to a large group at once.
You will need Access Level: SendHttp.
Load library using the following line:
use ElasticEmail::EmailsApi; use ElasticEmail::Object::EmailMessageData; use ElasticEmail::Object::EmailRecipient; use ElasticEmail::Object::EmailContent; use ElasticEmail::Object::BodyPart;
Get client instance, generate and use your API key (remember to check the required access level):
my $api_instance = ElasticEmail::EmailsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
First, you need to specify email details:
email recipients:
this example demonstrates merge fields usage, for each recipient {name}
will be changed to the recipient's name
email content:
body parts – in HTML, PlainText or in both from email – it needs to be your validated email address email subject
Find out more by checking our API's documentation.
my $email_message_data = ElasticEmail::Object::EmailMessageData->new(); # EmailMessageData | Email data my $recipient = ElasticEmail::Object::EmailRecipient->new(); $recipient->email('test@domain.com'); my $content = ElasticEmail::Object::EmailContent->new(); my $body = ElasticEmail::Object::BodyPart->new(); $body->content('body content'); my $body_content_type = 'HTML'; $body->content_type($body_content_type); $content->body([$body]); $content->reply_to('test@domain.com'); $content->from('test@domain.com'); $content->subject('template subject'); $content->template_name('template name'); $email_message_data->recipients([$recipient]); $email_message_data->content($content);
And finally, call emails_post
method from the API to send an email:
my $result = $api_instance->emails_post(email_message_data => $email_message_data);
Managing Campaigns
Add Campaign
To add your first campaign using the Perl library, you will need Access Level: ModifyCampaigns. Mind that when using Elastic Email, when you send an email to any group of contacts, we call it a “campaign”.
To add a campaign, put the following code into your file.
Load library using the following line:
use ElasticEmail::Object::Campaign; use ElasticEmail::Object::CampaignStatus; use ElasticEmail::Object::CampaignTemplate; use ElasticEmail::Object::CampaignRecipient; use ElasticEmail::Object::CampaignOptions; use ElasticEmail::CampaignsApi;
Get client instance, generate and use your API key (remember to check the required access level):
my $api_instance = ElasticEmail::CampaignsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create an example campaign object:
Name: defines the campaign name by which you can identify it later
Recipients: define your audience
Content: define your message details
Status: define the status in which the campaign should be created
Find out more by checking our API's documentation.
my $name = 'new campaign name'; my $recipient = ElasticEmail::Object::CampaignRecipient->new(); $recipient->segment_names(['segment name']); my $options = ElasticEmail::Object::CampaignOptions->new(); my $template = ElasticEmail::Object::CampaignTemplate->new(); $template->template_name('template name'); $template->reply_to('test@domain.com'); $template->from('test@domain.com'); $template->subject('subject'); my $campaign = ElasticEmail::Object::Campaign->new(); # Campaign | JSON representation of a campaign $campaign->name($name); $campaign->options($options); $campaign->recipients($recipient); $campaign->content([$template]);
Create an instance of CampaignsApi that will be used to create a campaign.
my $result = $api_instance->campaigns_post(campaign => $campaign);
Load Campaign List
To load details about a campaign list in your account using the Perl library, you will need Access Level: ViewCampaigns. Mind that when using Elastic Email, when you send an email to any group of contacts, we call it a “campaign”.
To load a campaign, put the following code into your file.
Load library using the following line:
use ElasticEmail::CampaignsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::CampaignsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
The only thing you need to specify is a campaign name
Find out more by checking our API's documentation.
You can add search, offset and limit parameters.
my $search = ""; # string | Text fragment used for searching in Campaign name (using the 'contains' rule) my $offset = 20; # int | How many items should be returned ahead. my $limit = 100; # int | Maximum number of returned items.
And finally, call campaigns_get
method from the API to get a campaign:
my $result = $api_instance->campaigns_get(search => $search, offset => $offset, limit => $limit);
Update Campaign
To update existing campaigns in your account using the Perl library, you will need Access Level: ModifyCampaigns. Mind that when using Elastic Email, when you send an email to any group of contacts, we call it a “campaign”.
To update a campaign, put the following code into your file.
Load library using the following line:
use ElasticEmail::CampaignsApi; use ElasticEmail::Object::Campaign; use ElasticEmail::Object::CampaignRecipient; use ElasticEmail::Object::CampaignTemplate; use ElasticEmail::Object::CampaignOptions;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::CampaignsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Create a whole campaign object that you want to put in place of a current version:
- Name: defines campaign name by which you can identify it later
- Recipients: define your audience
- Conent: define your message details
- Status: define status in which campaign should be created
Find out more by checking our API's documentation.
Send will be triggered immediately or postponed, depending on given options. Because we define Status
as Draft
, so in this case it will be postponed and campaign will be added to drafts.
my $name = "campaign name"; # string | Name of Campaign to update my $campaign = ElasticEmail::Object::Campaign->new(); # Campaign | JSON representation of a campaign $campaign->name('new campaign name'); my $recipient = ElasticEmail::Object::CampaignRecipient->new(); @array = ("recipient list name 1", "recipient list name 2"); $recipient->list_names(@array); $campaign->recipients($recipient); my $options = ElasticEmail::Object::CampaignOptions->new(); my $template = ElasticEmail::Object::CampaignTemplate->new(); $template->template_name('template name'); $template->reply_to('test@domain.com'); $template->from('test@domain.com'); $template->subject('subject'); $campaign->options($options); $campaign->content([$template]);
And finally, call campaigns_by_name_put
method from the API to update a campaign:
my $result = $api_instance->campaigns_by_name_put(name => $name, campaign => $campaign);
Delete Campaign
To delete an existing campaign, you will need Access Level: ModifyCampaigns. Mind that when using Elastic Email, when you send an email to any group of contacts, we call it a “campaign”.
To delete a campaign, put the following code into your file.
Load library using the following line:
use ElasticEmail::CampaignsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::CampaignsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
The only thing you need to specify is a campaign nameFind out more by checking our API's documentation.
my $name = "campaign name"; # string | Name of Campaign to delete
And finally, call campaigns_by_name_delete
method from the API to delete campaign:
$api_instance->campaigns_by_name_delete(name => $name);
Tracking
In this section, we will walk you through the steps of managing actions related to email tracking. You will get to know how to load delivery and campaign statistics from your account using the Perl library.
Load Statistics
To load delivery statistics from your account, you will need Access Level: ViewReports.
Put the following code into your file.
Load library using the line:
use ElasticEmail::StatisticsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::StatisticsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
First, you need to specify a date range:
- from date
- to date – optional
Find out more by checking our API's documentation.
my $from = DateTime->from_epoch(epoch => str2time('2021-02-01T11:00:00')); # DateTime | Starting date for search in YYYY-MM-DDThh:mm:ss format. my $to = DateTime->from_epoch(epoch => str2time('2022-03-01T11:00:00')); # DateTime | Ending date for search in YYYY-MM-DDThh:mm:ss format.
And finally, call statistics_get
method from the API to fetch statistics:
my $result = $api_instance->statistics_get(from => $from, to => $to);
Load Channel Statistics
To load statistics for each channel from your account, you will need Access Level: ViewChannels.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::StatisticsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::StatisticsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Channels statistics reponse is paginated you need to specfiy pagination options:
- limit – maximum returned items,
limit = 0
means to return everything till the end of the list - offset – how many items should be skipped from begging
Eg. to return second page of elements paginated 20 elements per page specify pagination options as follows
{ limit: 20, offset: 0, };
Find out more by checking our API's documentation.
Let's fetch everything:
my $limit = 100; # int | Maximum number of returned items. my $offset = 20; # int | How many items should be returned ahead.
And finally, call statistics_channels_get
method from the API to fetch statistics:
my $result = $api_instance->statistics_channels_get(limit => $limit, offset => $offset);
Load Campaigns Stats
To load statistics for each email campaign from your account, you will need Access Level: ViewChannels.
Put the following code into your file.
Load library using the following line:
use ElasticEmail::StatisticsApi;
Get client instance, generate and use your API key (remember to check a required access level):
my $api_instance = ElasticEmail::StatisticsApi->new( api_key => {'X-ElasticEmail-ApiKey' => 'YOUR_API_KEY'}, );
Campaigns statistics reponse is paginated you need to specfiy pagination options:
- limit – maximum returned items,
limit = 0
means to return everything till the end of the list - offset – how many items should be skipped from begging
Eg. to return second page of elements paginated 20 elements per page specify pagination options as follows
{ limit: 20, offset: 0, };
Find out more by checking our API's documentation.
Let's fetch everthing:
my $limit = 100; # int | Maximum number of returned items. my $offset = 20; # int | How many items should be returned ahead.
And finally, call statistics_campaigns_get
method from the API to fetch statistics:
my $result = $api_instance->statistics_campaigns_get(limit => $limit, offset => $offset);
Ready to get started?
Tens of thousands of companies around the world already send their emails with Elastic Email. Join them and discover your own email superpowers.