Elastic Email Typescript Angular API Library
Introduction
When you integrate Elastic Email with your application, you can interact with your Elastic Email account directly by our API. You can easily integrate your email flow, manage your contacts and templates, send emails, and track email statistics directly from your app.
This page will help you easily integrate with Elastic Email using the Typescript Angular library. You can find our whole downloadable Typescript Angular repository on GitHub. You can also use our comprehensive API documentation.
Elastic Email API v4 uses REST architecture, which allows you to perform various code actions, including directly through your app.
On this page, you will find how to authenticate your application and what the requirements for the integration are. You will be also provided with a quick start guide on how to start using API, followed by code samples.
Authentication
To provide valid authentication and start using our API, you will need an API key. To generate your API key, enter settings on your Elastic Email account and go to Settings -> Manage API Keys -> Create or you can also click on the link:
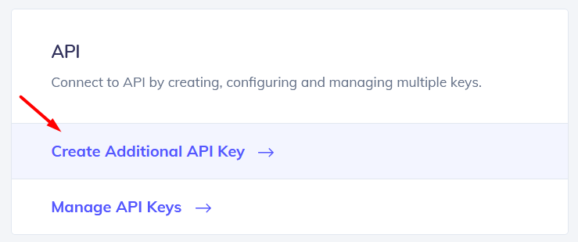
At this point, you can set custom permissions and optional access for your API key.
Security tip: The restriction forces the API Key to work only with an IP or IP range that will be specified in this field.
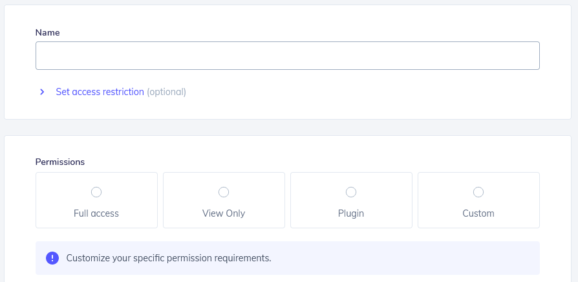
Once you create your API key, keep it safe as it will be used for every API call you make in order to identify you and confirm your account’s credentials. You can create either up to 15 or an unlimited amount of API keys based on your pricing plan.
Your API key should be sent inside the header with the parameter name ‘X-ElasticEmail-ApiKey’ and your API key as a value.
Installation and Usage
Installation
Our official downloadable Typescript Angular library is available on GitHub.
To install the required dependencies and to build the typescript sources run:
npm install npm run build
publishing
First, build the package then run npm publish dist
(don't forget to specify the dist folder!)
without publishing
First, build the package and change the directory to the dist folder. Next run npm pack
(make sure to switch directories first!)
consuming
Navigate to the folder of your consuming project and run one of the next commands.
published:
npm install elasticemail-angular@4.0.21 --save

without publishing (not recommended):
npm install PATH_TO_GENERATED_PACKAGE/elasticemail-angular-4.x.xx.tgz --save

It's important to take the tgz file, otherwise you'll get trouble with links on windows
using npm link
:
In PATH_TO_GENERATED_PACKAGE/dist:
npm link
In your project:
npm link elasticemail-angular
Note for Windows users: The Angular CLI has troubles to use linked npm packages. Please refer to this issue angular/angular-cli#8284 for a solution / workaround. Published packages are not effected by this issue.
Usage
In your Angular project:
// without configuring providers import { ApiModule } from 'elasticemail-angular'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ imports: [ ApiModule, // make sure to import the HttpClientModule in the AppModule only, // see https://github.com/angular/angular/issues/20575 HttpClientModule ], declarations: [ AppComponent ], providers: [], bootstrap: [ AppComponent ] }) export class AppModule {}

// configuring providers import { ApiModule, Configuration, ConfigurationParameters } from 'elasticemail-angular'; export function apiConfigFactory (): Configuration { const params: ConfigurationParameters = { // set configuration parameters here. } return new Configuration(params); } @NgModule({ imports: [ ApiModule.forRoot(apiConfigFactory) ], declarations: [ AppComponent ], providers: [], bootstrap: [ AppComponent ] }) export class AppModule {}

// configuring providers with an authentication service that manages your access tokens import { ApiModule, Configuration } from 'elasticemail-angular'; @NgModule({ imports: [ ApiModule ], declarations: [ AppComponent ], providers: [ { provide: Configuration, useFactory: (authService: AuthService) => new Configuration( { basePath: environment.apiUrl, accessToken: authService.getAccessToken.bind(authService) } ), deps: [AuthService], multi: false } ], bootstrap: [ AppComponent ] }) export class AppModule {}

import { DefaultApi } from 'elasticemail-angular'; export class AppComponent { constructor(private apiGateway: DefaultApi) { } }

Note: The ApiModule is restricted to being instantiated once app wide. This is to ensure that all services are treated as singletons.
Using Multiple OpenAPI files / APIs / ApiModules
In order to use multiple ApiModules
generated from different OpenAPI files, you can create an alias name when importing the modules in order to avoid naming conflicts:
import { ApiModule } from 'my-api-path'; import { ApiModule as OtherApiModule } from 'my-other-api-path'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ imports: [ ApiModule, OtherApiModule, // make sure to import the HttpClientModule in the AppModule only, // see https://github.com/angular/angular/issues/20575 HttpClientModule ] }) export class AppModule { }

Set service base path
If different than the generated base path, during app bootstrap, you can provide the base path to your service.
import { BASE_PATH } from 'elasticemail-angular'; bootstrap(AppComponent, [ { provide: BASE_PATH, useValue: 'https://your-web-service.com' }, ]);

or
import { BASE_PATH } from 'elasticemail-angular'; @NgModule({ imports: [], declarations: [ AppComponent ], providers: [ provide: BASE_PATH, useValue: 'https://your-web-service.com' ], bootstrap: [ AppComponent ] }) export class AppModule {}

Using @angular/cli
First extend your src/environments/*.ts
files by adding the corresponding base path:
export const environment = { production: false, API_BASE_PATH: 'http://127.0.0.1:8080' };

In the src/app/app.module.ts:
import { BASE_PATH } from 'elasticemail-angular'; import { environment } from '../environments/environment'; @NgModule({ declarations: [ AppComponent ], imports: [ ], providers: [{ provide: BASE_PATH, useValue: environment.API_BASE_PATH }], bootstrap: [ AppComponent ] }) export class AppModule { }

Quick start guide
In this section, we will tell you the steps to start sending emails with our email API.
1. Register a free account
Register and activate your Elastic Email account to get access to our product.
2. Verify your domain
Follow the instructions on our settings page to verify your domain and start sending with us.
3. Create an API Key
Go to your setting to generate an API Key to add to your code later.
4. Install our libraries
Install our Typescript Angular library as explained in the Installation and usage section.
5. Send your first email with API
Now it’s time to send your first test email with API to make sure that everything is set up correctly after downloading the library and the authentication process.
In app.module.ts:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import {HttpClientModule} from '@angular/common/http'; import { AppComponent } from './app.component'; import { ApiModule } from 'elasticemail-angular'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, HttpClientModule, ApiModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
In app.component.ts:
import { Component, inject, OnInit } from '@angular/core'; import {HttpClient} from '@angular/common/http'; import { BodyContentType, BodyPart, Configuration, EmailContent, EmailTransactionalMessageData, TransactionalRecipient, EmailsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ // Creation of objects to build your email var bodyType: BodyContentType = 'PlainText'; var content: BodyPart = {ContentType: bodyType, Content: "Test", Charset: "utf-8"}; var body: EmailContent = {Body: [content], From: "<from address>", Subject: "Test123"}; var recipient: TransactionalRecipient = {To: ["<to address>"]}; var email: EmailTransactionalMessageData = {Recipients: recipient, Content: body}; // Creation of the Configuration object for Authentication var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; // Create the Service object with your http client, base path, and authentication var serv = new EmailsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.emailsTransactionalPost(email).subscribe((res) => { // Display the results in the console and on a web page (Both optional) console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
6. Sending without library
Although it is recommended to use our library, you can still send emails through our API without it once you authenticate. This Example code uses HttpClient.
In app.module.ts:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import {HttpClientModule} from '@angular/common/http'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
In app.component.ts:
import { Component, inject, OnInit } from '@angular/core'; import {HttpClient} from '@angular/common/http'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(){ this.SendCall(); } SendCall(){ // Create a json object body var email: string = JSON.stringify({ "Recipients": { "To": [ "<to address>" ] }, "Content": { "Body": [ { "ContentType": "HTML", "Content": "Lorem ali", "Charset": "utf-8" } ], "From": "<from address>", "Subject": "Hello!" } }); // Set the headers and url of your API call let url = "https://api.elasticemail.com/v4/emails/transactional"; let headers = {'X-ElasticEmail-ApiKey':'<your API key>', 'Content-Type':'application/json'}; // Send the API call and view the response this.httpClient .post(url, email, {headers}) .subscribe((res) => { // Both of these are optional console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Code Samples
Once you have sent the test email with our API, you can start sending transactional emails and perform various operations directly through your app. We have prepared for you some code samples for most essential actions, including managing contacts and lists, creating templates, and sending and tracking emails. Thanks to them, your integration with our API will be an even easier and more pleasant experience.
In case any of the following snippets are changed in the meantime, the most recent version of this API Library is available on GitHub.
The following code sample is for your app.module.ts file used in all of the samples below:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import {HttpClientModule} from '@angular/common/http'; import { AppComponent } from './app.component'; import { ApiModule } from 'elasticemail-angular'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, HttpClientModule, ApiModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Sending Emails
In this section, we will tell you how you can start sending emails using the Typescript Angular library. You will get to know how to send bulk and transactional emails.
Send Transactional Emails
To send transactional emails, you will need Access Level: SendHttp.
When sending transactional emails all recipients will be visible to one another and you will be able to add CC or BCC addresses.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import {HttpClient} from '@angular/common/http'; import { BodyContentType, BodyPart, Configuration, EmailContent, EmailTransactionalMessageData, TransactionalRecipient, EmailsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ // Creation of objects to build your email var bodyType: BodyContentType = 'PlainText'; var content: BodyPart = {ContentType: bodyType, Content: "Test", Charset: "utf-8"}; var body: EmailContent = {Body: [content], From: "<from address>", Subject: "Test123"}; var recipient: TransactionalRecipient = {To: ["<to address>"]}; var email: EmailTransactionalMessageData = {Recipients: recipient, Content: body}; // Creation of the Configuration object for Authentication var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; // Create the Service object with your http client, base path, and authentication var serv = new EmailsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.emailsTransactionalPost(email).subscribe((res) => { // Display the results in the console and on a web page (Both optional) console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
The creation of the different variables used for the email content
use lines 21-25:
- The Body Content Type on line 21 sets the content type, plaintext or HTML
- The Body Part made on line 22 contains the body content type, the content of your email, and the Charset you want to use
- The Email Content on line 23 will contain a list of your body parts, the sender address, and subject of your email
- The Transactional Recipient on line 24 will contain a list of emails that you wish to send the email to
- Finally, the Email Transactional Message Data on line 25 will contain the email content and transactional recipient you made
Create your configuration and set your API key and the base path
using lines 27-29
The service object will be made with line 31
Finally, send your email and view the results using lines 32-35
Viewing the results is not mandatory, lines 33-34 are optional
Find out more by checking our API's documentation
Send Bulk Emails
You will need Access Level: SendHttp.
When sending bulk emails recipients will not be visible to one another and you will not be able to CC or BCC other addresses.
Put the following code to your file:
import { Component, inject, OnInit } from '@angular/core'; import {HttpClient} from '@angular/common/http'; import { BodyContentType, BodyPart, Configuration, EmailContent, EmailMessageData, EmailRecipient, EmailsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var bodyType: BodyContentType = 'PlainText'; var content: BodyPart = {ContentType: bodyType, Content: "Test", Charset: "utf-8"}; var body: EmailContent = {Body: [content], From: "<from address>", Subject: "Test123"}; var recipient: EmailRecipient = {Email: "<to address>"}; var email: EmailMessageData = {Recipients: [recipient], Content: body}; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new EmailsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.emailsPost(email).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
The creation of the different variables used for the email content
use lines 21-25:
- The Body Content Type on line 21 sets the content type,
plaintext or HTML - The Body Part made on line 22 contains the body content type, the content of your email, and the Charset you want to use
- The Email Content on line 23 will contain a list of your body parts, the sender address, and subject of your email
- The Recipient on line 24 will contain the address of your recipient. To send to multiple people, you will have to make many of these objects
- Finally, the Email Message Data on line 25 will contain the email content and a list of recipients you made
Create your configuration and set your API key and the base path
using lines 27-29
The service object will be made with line 31
Finally, send your email and view the results using lines 32-35
Viewing the results is not mandatory, lines 33-34 are optional
Find out more by checking our API's documentation
Managing Campaigns
In this section, we will tell you how you can start sending and managing campaigns using the Typescript Angular library. Mind that when using Elastic Email, when you send an email to any group of contacts, we call it a "campaign".
Add Campaign
To add a campaign, you will need Access Level: ModifyCampaigns.
To add a campaign, put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Campaign, CampaignRecipient, CampaignsService, CampaignTemplate, Configuration } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var recipients: CampaignRecipient = {ListNames: ["<list name>"]}; var template: CampaignTemplate = {From: "<from address>", TemplateName: "<template name>", ReplyTo: "<reply to address>", Subject: "angular campaign"}; var campaign: Campaign = {Name: "angular campaign", Recipients: recipients, Content: [template]}; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new CampaignsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.campaignsPost(campaign).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
The creation of the objects used in the campaign use lines 21-23:
- The Recipients on line 21 will take an array of your list names that you want to send to
- The Campaign Template on line 22 will take most of the options, including the sender address, the template name, the reply to (optional), and the email subject
- Finally, the Campaign object on line 23 will take a campaign name, and the recipients and content objects you made previously
Create your configuration and set your API key and the base path
using lines 25-27
The service object will be made with line 29
Finally, create your campaign and view the results using lines 30-33
Viewing the results is not mandatory, lines 31-32 are optional
Find out more by checking our API's documentation
Load Campaign
To load details about a campaign list in your account, you will need Access Level: ViewCampaigns.
To load a campaign, put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { CampaignsService, Configuration } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var name = "<your campaign name>"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new CampaignsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.campaignsByNameGet(name).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Specify your campaign by campaign name on line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, load your campaign and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Update Campaign
To update existing campaigns in your account, you will need Access Level: ModifyCampaigns.
To update a campaign, put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Campaign, CampaignRecipient, CampaignsService, CampaignTemplate, Configuration } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var name = "Draft to Active"; var recipients: CampaignRecipient = {ListNames: ["<list name>"]}; var template: CampaignTemplate = {From: "<from address>", TemplateName: "<your template>", ReplyTo: "<reply to address>", Subject: "angular campaign"}; var campaign: Campaign = {Name: "angular campaign updated", Recipients: recipients, Content: [template]}; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new CampaignsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.campaignsByNamePut(name, campaign).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Select the campaign you would like to update by campaign name
using line 21
The creation of the objects used in the campaign use lines 22-24:
- The Recipients on line 22 will take an array of your list names that you want to send to
- The Campaign Template on line 23 will take most of the options, including the sender address, the template name, the reply to (optional), and the email subject
- Finally, the Campaign object on line 24 will take a campaign name, and the recipients and content objects you made previously
Create your configuration and set your API key and the base path
using lines 26-28
The service object will be made with line 30
Finally, update your campaign and view the results using lines 31-34
Viewing the results is not mandatory, lines 32-33 are optional
Find out more by checking our API's documentation
Delete Campaign
To delete an existing campaign, you will need Access Level: ModifyCampaigns.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { CampaignsService, Configuration } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var name = "<campaign to delete name>"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new CampaignsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.campaignsByNameDelete(name).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Specify your campaign by campaign name on line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, delete your campaign and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Tracking
In this section, we will walk you through the steps of managing actions related to email tracking. You will get to know how to load delivery and campaign statistics from your account using the Typescript Angular library.
Load Statistics
To Load delivery statistics from your account, you will need Access Level: ViewReports.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, StatisticsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var from = "2024-01-01"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<Your API Key>"}; var serv = new StatisticsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.statisticsGet(from).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Set the start date (yyyy-mm-dd) for the stats using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, load your statistics and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Load Channels Statistics
To load statistics for each channel from your account, you will need Access Level: ViewChannels.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, StatisticsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<Your API Key>"}; var serv = new StatisticsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.statisticsChannelsGet().subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Create your configuration and set your API key and the base path
using lines 21-23
The service object will be made with line 25
Finally, load your statistics and view the results using lines 26-29
Viewing the results is not mandatory, lines 27-28 are optional
Find out more by checking our API's documentation
Load Campaign Statistics
To load statistics for each email campaign from your account, you will need Access Level: ViewChannels.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, StatisticsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<Your API Key>"}; var serv = new StatisticsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.statisticsCampaignsGet().subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Create your configuration and set your API key and the base path
using lines 21-23
The service object will be made with line 25
Finally, load your statistics and view the results using lines 26-29
Viewing the results is not mandatory, lines 27-28 are optional
Find out more by checking our API's documentation
Managing Contacts
In this section, we will walk you through managing the contacts on your account using the Typescript Angular library.
Add Contacts
To Add contacts to your account, you will need Access Level: ModifyContacts
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ContactPayload, ContactsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var listname = "My List"; var contactPayload: ContactPayload = {Email: "<address to add>"}; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<Your API Key>"}; var serv = new ContactsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.contactsPost([contactPayload], [listname]).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Set the list name you want to add contacts to using line 21
Create a Contact Payload object with email you want to add using line 22
Create your configuration and set your API key and the base path
using lines 24-26
The service object will be made with line 28
Finally, add the contact and view the results using lines 29-32
Viewing the results is not mandatory, lines 30-31 are optional
Find out more by checking our API's documentation
Add Contacts Bulk
To Add contacts to your account, you will need Access Level: ModifyContacts
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ContactPayload, ContactsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void { this.SendCall(); } SendCall(): void{ var listname = "My List"; var contactList: ContactPayload[] = []; contactList.push({Email: "<contact address 1>"}); contactList.push({Email: "<contact address 2>"}); contactList.push({Email: "<contact address 3>"}); var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new ContactsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.contactsPost(contactList, [listname]).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Set the list name you want to add contacts to using line 21
Create a Contact Payload object array using line 23
Populate the list with your contact emails using lines 24-26
Create your configuration and set your API key and the base path
using lines 28-30
The service object will be made with line 32
Finally, add the contacts and view the results using lines 33-36
Viewing the results is not mandatory, lines 34-35 are optional
Find out more by checking our API's documentation
Delete Contacts
If you want to delete a contact, you will need Access Level: ModifyContacts.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ContactsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var address = "<address to delete>"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<Your API Key>"}; var serv = new ContactsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.contactsByEmailDelete(address).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Select the contact you want to delete by email using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, delete the contact and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Export Contacts
If you want to export the selected contact to a downloadable file, you will need Access Level: Export
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ContactsService, ExportFileFormats } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var fileFormat: ExportFileFormats = "Csv"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new ContactsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.contactsExportPost(fileFormat).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Select the file format you want to receive using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, export your contacts and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Managing Lists
In this section, we will walk you through managing your contact list on your account using the Typescript Angular library.
Add List
To Add a list, you will need Access Level: ModifyContacts.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ListPayload, ListsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var listPayload: ListPayload = {ListName: "Angular List"}; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new ListsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.listsPost(listPayload).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Create the list payload object using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, add your list and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Load List
To Load a list, you will need Access Level: ViewContacts.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ListsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var listName = "Angular List"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new ListsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.listsByNameGet(listName).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Specify the list by list name using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, load your list and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Delete List
To Remove a contact list from your account, you will need Access Level: ModifyContacts.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, ListsService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var listName = "Angular List"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; var serv = new ListsService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.listsByNameDelete(listName).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Specify the list by list name using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, delete your list and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Creating Templates
An email template is a body of email prepared and saved under a given name. In this section, you will get to know how to add and load email templates.
Add Template
To add a template, you will need Access Level: ModifyTemplates.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { BodyPart, Configuration, TemplatePayload, TemplatesService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var bodyPart: BodyPart = {ContentType: "HTML", Content: "<p>Test email content</p>", Charset: "utf-8"}; var templatePayload: TemplatePayload = {Name: "Angular Template", Body: [bodyPart]}; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<Your API Key>"}; var serv = new TemplatesService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.templatesPost(templatePayload).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Create the template Body Part object using line 21. This will contain the Content Type, the Content of your email, and the charset
Also create the Template Payload object with your body part object and template name using line 22
Create your configuration and set your API key and the base path
using lines 24-26
The service object will be made with line 28
Finally, create your template and view the results using lines 29-32
Viewing the results is not mandatory, lines 30-31 are optional
Find out more by checking our API's documentation
Load Template
To load existing template details, you will need Access Level: ViewTemplates.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, TemplatesService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ var name = "Angular Template"; var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "your API key"}; var serv = new TemplatesService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.templatesByNameGet(name).subscribe((res) => { console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Specify the template by template name using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, load your template and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Delete Template
To delete a template, you will need Access Level: ModifyTemplates.
Put the following code into your file:
import { Component, inject, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Configuration, TemplatesService } from 'elasticemail-angular'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit{ title = 'libtest15'; httpClient = inject(HttpClient); data: any; ngOnInit(): void{ this.SendCall(); } SendCall(): void{ // Creation of a variable to specify template name var name = "Angular Template"; // Creation of the Configuration object for Authentication var config = new Configuration(); config.basePath = "https://api.elasticemail.com/v4"; config.credentials = {apikey: "<your API key>"}; // Create the Service object with your http client, base path, and authentication var serv = new TemplatesService(this.httpClient, "https://api.elasticemail.com/v4", config); serv.templatesByNameDelete(name).subscribe((res) => { // Display the results in the console and on a web page (Both optional) console.log(JSON.stringify(res)); this.data = JSON.stringify(res); }); } }
Load the library and other dependencies using lines 1-3
Mark the class as a component with lines 5-9
Set the webpage title, inject the http client, and create the data field using lines 12-14. The data field is optional, but can be shown on the webpage
ngOnInit is used to call the function on page load in this example
using lines 16-18
Specify the template by template name using line 21
Create your configuration and set your API key and the base path
using lines 23-25
The service object will be made with line 27
Finally, delete your template and view the results using lines 28-31
Viewing the results is not mandatory, lines 29-30 are optional
Find out more by checking our API's documentation
Limitations
The maximum email size of your entire message or message + attachment cannot exceed 20MB.
The attachment format is the file’s content as a byte array or a Base64 string.
The maximum number of recipients has no limit for one campaign. It depends on the pricing plan.
The API has a limit of 20 concurrent connections and a hard timeout of 600 seconds per request.
Ready to get started?
Tens of thousands of companies around the world already send their emails with Elastic Email. Join them and discover your own email superpowers.